To start let's define the following
user=>(defn sort-map [m] (apply sorted-map (apply concat m)))
That's right. A function to cast hash-map to tree-maps.
user=>(sort-map {3 :a 1 :c 2 :b})
{1 :c, 2 :b, 3 :a}
Why would I do that? Because I now have a way of making subsets based on the keys.
user=>(keys-pred take-while #(< 2 %) (sort-map {3 :a 1 :c 2 :b}))
{1 :c}
user=>(keys-pred drop-while #(< 2 %) (sort-map {3 :a 1 :c 2 :b}))
{2 :b, 3 :a}
So, when would this have an application?
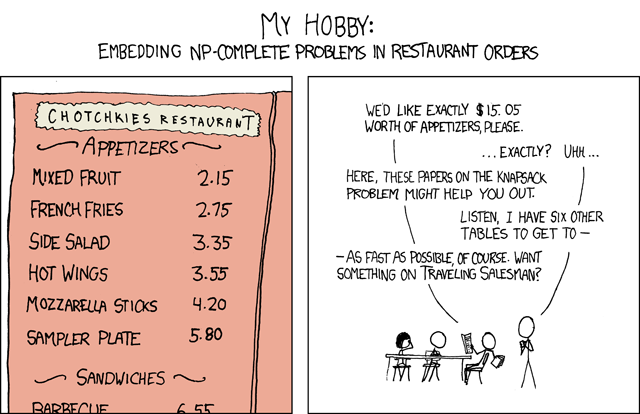
Let's define the following map
And there you go, a use for take/drop while with maps :)
No comments:
Post a Comment